Integrate Google Firebase to Handle User Authentication
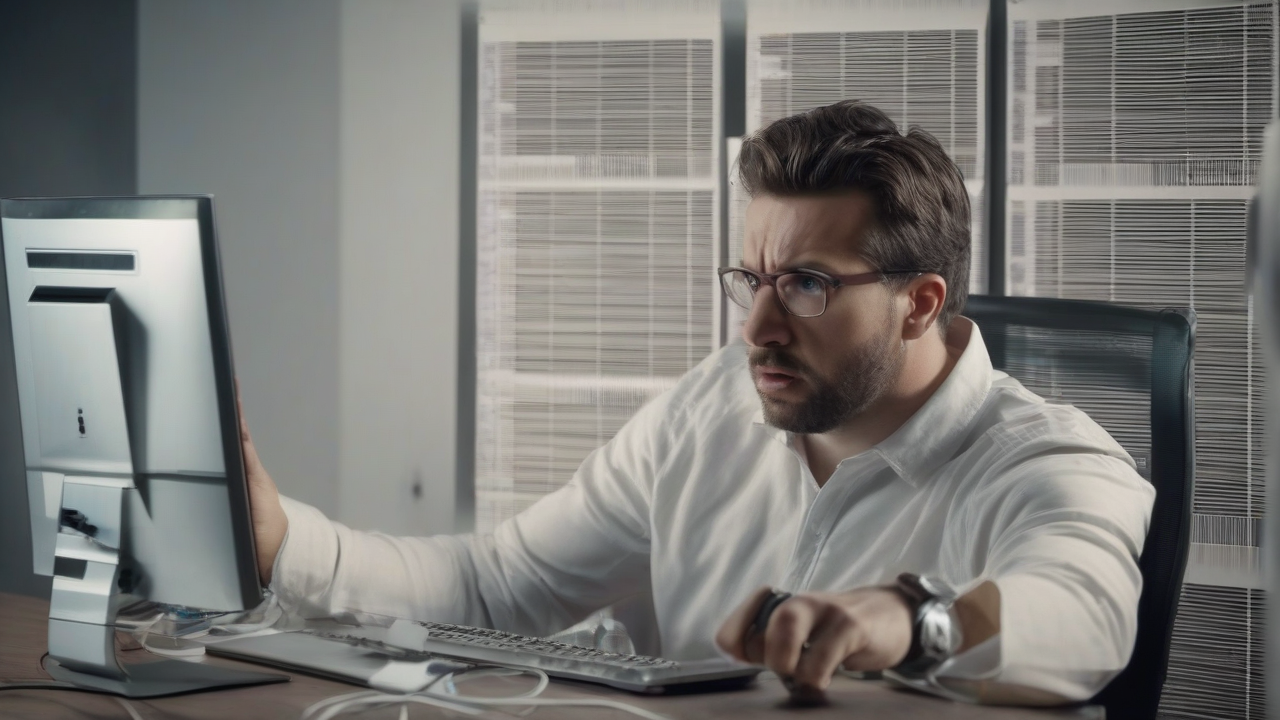
Overview
This comprehensive guide delves into the intricacies of integrating Google Firebase to handle user authentication within your applications. We will explore various authentication methods, security best practices, and advanced techniques to build a secure and robust user experience. From the initial setup to implementing advanced features, this guide provides a step-by-step approach suitable for developers of all skill levels. We will cover everything from basic email/password authentication to more advanced methods such as social logins and phone authentication, ensuring your application caters to a wide range of user preferences and security needs.
Setting Up Your Firebase Project
Before you begin integrating Firebase into your application, you need to create a Firebase project and configure it properly. This section outlines the steps involved in setting up your Firebase project and configuring it for authentication.
1. Creating a Firebase Project
The first step is to create a Firebase project. Go to the Firebase Console and click on "Add project". You'll need to provide a project name and select your country/region. Follow the instructions to create your project. This process usually involves accepting the Firebase terms of service.
2. Registering Your App
Once your project is created, you'll need to register your app within the Firebase project. Select your app platform (Android, iOS, web, etc.). You will be prompted to download a google-services.json
file (for Android) or a GoogleService-Info.plist
file (for iOS). These files contain your app's unique configuration details necessary for communication with your Firebase project. Keep these files secure; they should not be committed to a public repository.
3. Installing the Firebase SDK
The next crucial step is to install the Firebase SDK into your application. The specific method depends on your application platform. For example, for web applications, you'll typically include a script tag in your HTML file referencing the Firebase JavaScript SDK. For mobile apps (Android and iOS), you'll need to add the necessary dependencies to your project's build files using your platform's package manager (Gradle for Android, CocoaPods or Swift Package Manager for iOS). Detailed instructions for each platform are available in the official Firebase documentation.
4. Enabling Authentication Methods
Within the Firebase console, navigate to the "Authentication" section. Here, you'll find options to enable various authentication methods, such as email/password, phone authentication, Google Sign-in, Facebook Sign-in, Twitter Sign-in, GitHub Sign-in, and more. Enable the methods you want your users to be able to use to sign in to your application. This flexibility allows you to offer users various convenient login options.
Email/Password Authentication
Email/password authentication is a fundamental authentication method. It involves users creating accounts using their email addresses and chosen passwords. This section will guide you through implementing email/password authentication using Firebase.
1. Creating a User
To create a new user account using email and password, you'll use the createUserWithEmailAndPassword()
method provided by the Firebase SDK. This method takes the user's email and password as arguments and creates a new user account in your Firebase project. Ensure to handle potential errors, such as invalid email addresses or weak passwords.
2. Signing In a User
To sign in an existing user, you'll utilize the signInWithEmailAndPassword()
method. This method also takes the email and password as arguments and verifies them against your Firebase project's user database. Remember to handle authentication errors gracefully, providing informative feedback to the user.
3. Password Reset
Providing a password reset functionality enhances user experience and security. Firebase offers the sendPasswordResetEmail()
method. This method sends a password reset email to the user's registered email address, allowing them to reset their password if they forget it.
4. Handling Authentication State
Efficiently managing authentication state is crucial for a smooth user experience. Use Firebase's authentication state listeners to monitor the user's login status. This allows you to dynamically update your application's UI based on whether a user is logged in or logged out. This provides a seamless transition between different parts of your application based on authentication status.
Social Logins (Google, Facebook, etc.)
Integrating social logins enhances user experience by allowing users to sign in using their existing social media accounts. This section details how to integrate social logins into your application using Firebase.
1. Enabling Social Logins in Firebase
As mentioned earlier, enable the desired social login providers (Google, Facebook, Twitter, etc.) within the Firebase console under the "Authentication" section. You'll need to configure the necessary API keys and settings for each provider, which usually involves creating an app within the respective social media platform.
2. Implementing Social Login in Your App
The Firebase SDK provides methods to handle authentication requests from various social login providers. Each provider has specific methods to initiate the sign-in process. For example, Google Sign-in will initiate a pop-up window where the user will need to sign in with their Google credentials. Upon successful authentication, Firebase will return a user object containing user information that you can use to personalize the user experience within your application.
3. Handling Social Login Responses
After a user initiates a social login, your app needs to handle the response appropriately. The SDK will provide a callback function that contains the user's information and authentication status. This information includes the user's ID, name, email address, and other profile details. Handle potential errors during the login process, providing informative feedback to the user if something goes wrong.
Phone Authentication
Phone authentication provides an alternative login method using users' phone numbers. This is particularly useful in regions with limited internet access or for users who prefer not to use email or social media accounts.
1. Enabling Phone Authentication
In the Firebase console, ensure that phone authentication is enabled. This may require enabling specific services or APIs. You may also need to configure your project to work with your specific country codes, which are necessary to properly manage phone numbers.
2. Sending Verification Codes
Use the Firebase SDK to send a verification code to the user's phone number via SMS. You'll need to specify the phone number in the correct international format (including the country code). The Firebase SDK will handle the actual communication with the user's mobile network.
3. Verifying Verification Codes
Once the user provides the verification code, use the Firebase SDK to verify it against the code sent previously. Upon successful verification, the user will be signed in. Ensure that you handle invalid or expired codes gracefully, providing appropriate feedback to the user.
4. Handling Phone Authentication Errors
Robust error handling is paramount for a positive user experience. Implement comprehensive error handling to catch issues such as invalid phone numbers, incorrect verification codes, network errors, or API limitations. Provide clear and informative error messages to guide users through the process.
Security Rules
Security rules are crucial for protecting your application's data and ensuring only authorized users can access sensitive information. This section highlights the importance of properly configuring Firebase security rules for authentication.
1. Defining Security Rules
Firebase security rules are written in a custom language that allows you to define access control for your data. These rules determine which users (or groups of users) have permission to read, write, or modify data in your Firebase database and storage. The rules are defined in a JSON-like format within the Firebase console.
2. Authentication-Based Rules
You can create security rules that are based on the user's authentication state. For example, you can define rules that only allow authenticated users to access specific parts of your database or storage. This prevents unauthorized access to sensitive data.
3. Role-Based Access Control (RBAC)
Implement role-based access control to grant different levels of access to different groups of users. This allows you to create user roles, such as "admin," "editor," and "viewer," each with different permissions. This granular control enhances the security and maintainability of your application.
4. Custom Claims
Firebase allows you to add custom claims to users' authentication tokens. Custom claims can be used to store additional user information, such as user roles or permissions. You can then use these custom claims in your security rules to further refine access control based on specific user attributes. This adds another layer of security and customization to your access controls.
Advanced Techniques and Best Practices
This section covers some advanced techniques and best practices to optimize your Firebase authentication implementation.
1. User Profiles
Extend the basic user profiles provided by Firebase by adding custom user data to your application. Store additional information, such as user names, profile pictures, and preferences, in a separate database collection. This allows you to personalize the user experience and provide tailored functionality to your users.
2. Email Verification
Enhance security by requiring email verification. When a user registers, send a verification email to their registered address. This email should contain a link or code that the user needs to click or input to verify their email address. This additional step helps prevent the creation of fake accounts.
3. Two-Factor Authentication (2FA)
Implement 2FA for enhanced security. This involves requiring users to provide a second form of authentication, such as a one-time password (OTP) sent to their phone number or a code from an authentication app. This significantly improves the security of your application, making it more resistant to unauthorized access.
4. Secure Password Storage
Never store passwords directly in your database. Firebase handles password hashing and salting automatically, ensuring that passwords are stored securely and cannot be easily deciphered. Always leverage the security features provided by Firebase to maintain high security standards.
5. Handling User Sessions
Implement proper session management. Use appropriate tokens and expiration times to manage user sessions effectively. Refresh tokens when necessary and automatically log users out after a period of inactivity. This ensures that only active users are logged in to your application.
6. Logging and Monitoring
Implement comprehensive logging and monitoring to track user authentication events. Monitor for any unusual activity or potential security breaches. Utilize Firebase's monitoring tools to track login attempts, successful logins, failed logins, and password reset requests. This provides valuable insights into the security and usage patterns of your application.
7. Testing and Debugging
Thoroughly test your authentication implementation to identify and fix bugs. Use Firebase's testing tools and simulate different user scenarios to ensure that your authentication system is functioning as expected. Implement comprehensive error handling to prevent crashes and unexpected behavior.
Integrating Firebase Authentication with Other Firebase Services
Firebase's strength lies in its interconnected services. This section explores how to integrate Firebase Authentication with other services, such as Firestore and Cloud Storage.
1. Firestore Security Rules
Use Firebase Authentication with Firestore security rules. Control access to your Firestore database based on the user's authentication status and roles. This ensures that only authenticated users can access the data that they are permitted to see.
2. Cloud Storage Security Rules
Similarly, you can integrate Firebase Authentication with Cloud Storage. Configure security rules to determine which users have access to files stored in Cloud Storage. This protects your files from unauthorized access or modification.
3. Realtime Database Security Rules
Firebase's Realtime Database also supports integration with Firebase Authentication. Control access to your Realtime Database based on the user's authentication credentials, ensuring data protection and integrity.
Troubleshooting Common Issues
This section provides solutions to some common issues encountered during the integration of Firebase Authentication.
1. Authentication Errors
Handle authentication errors gracefully. Provide informative error messages to users so they understand why they cannot sign in. Thoroughly test various error scenarios and ensure that your application responds appropriately to unexpected situations.
2. Security Rule Conflicts
Resolve any conflicts between your security rules and your authentication implementation. Make sure that your rules allow for the expected user behavior. Carefully review and test your security rules to prevent any unexpected issues.
3. SDK Issues
Address issues related to the Firebase SDK. Refer to the official Firebase documentation for the latest updates, bug fixes, and best practices. Ensure that you use compatible versions of the SDK to avoid conflicts or issues.
4. Data Synchronization Problems
If you are experiencing data synchronization problems, review your data models and ensure that your data structures are consistent across all your Firebase services. Verify that your security rules correctly manage data access and updates.
Conclusion
Integrating Google Firebase for user authentication offers a robust and scalable solution for your applications. By leveraging Firebase's various authentication methods, security features, and best practices, you can create secure and user-friendly applications. This guide has provided a comprehensive overview of the process, covering setup, various authentication methods, security rules, and advanced techniques. Remember to continuously monitor your authentication implementation for potential security vulnerabilities and proactively address them to maintain the integrity and security of your applications. As Firebase continuously evolves, staying updated with the latest features and best practices will ensure that your authentication remains secure and efficient.